고려대 컴퓨터정보학과
Operating System Programming Assignment 마지막~!
Dining Philosopher
사실 내가 백프로 짠 쏘스도 아니고
마지막이기도 하고
걍 소스코드 전부다 공개
dp.h 소스코드
#ifndef __Dining_Philosopher_h
#define __Dining_Philosopher_h
#define MAXLIST 256
#define MAXNAME 32
class Dining_Philosopher
{
private:
int m_id;
#endif
dp.cpp 소스코드
Dining_Philosopher::~Dining_Philosopher(void)
{
}
void Dining_Philosopher::print()
{
printf("philosopher DI: %3d\n", m_id);
}
void Dining_Philosopher::eat()
{
int eat_time = 1 + rand() % 10;
void Dining_Philosopher::think()
{
int think_time = 1 + rand() % 10;
jhs.cpp 소스코드 (메인파일)
DWORD WINAPI WriteToDatabase( LPVOID );
이건 실행화면
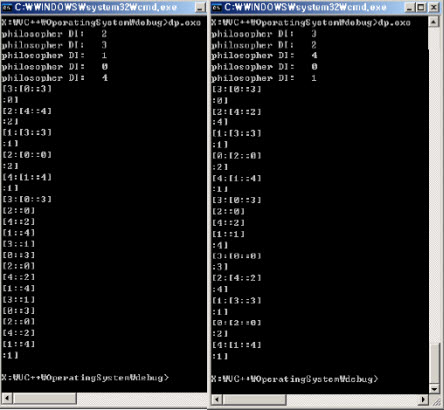
Operating System Programming Assignment 마지막~!
Dining Philosopher
사실 내가 백프로 짠 쏘스도 아니고
마지막이기도 하고
걍 소스코드 전부다 공개
dp.h 소스코드
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
#include <math.h>
#include <tchar.h>
#include <windows.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
#include <math.h>
#include <tchar.h>
#include <windows.h>
#ifndef __Dining_Philosopher_h
#define __Dining_Philosopher_h
#define MAXLIST 256
#define MAXNAME 32
class Dining_Philosopher
{
private:
int m_id;
public:
Dining_Philosopher(int id);
~Dining_Philosopher();
Dining_Philosopher(int id);
~Dining_Philosopher();
void print();
void eat();
void think();
void eat();
void think();
protected:
};
#endif
dp.cpp 소스코드
#include "dp.h"
Dining_Philosopher::Dining_Philosopher(int id)
{
memset(this, 0, sizeof(Dining_Philosopher) );
srand( (unsigned int)time(NULL) );
{
memset(this, 0, sizeof(Dining_Philosopher) );
srand( (unsigned int)time(NULL) );
this->m_id = id;
this->print();
}
this->print();
}
Dining_Philosopher::~Dining_Philosopher(void)
{
}
void Dining_Philosopher::print()
{
printf("philosopher DI: %3d\n", m_id);
}
void Dining_Philosopher::eat()
{
int eat_time = 1 + rand() % 10;
printf("[%1d:", m_id);
Sleep( eat_time );
printf(":%1d]\n", m_id);
}
Sleep( eat_time );
printf(":%1d]\n", m_id);
}
void Dining_Philosopher::think()
{
int think_time = 1 + rand() % 10;
Sleep( think_time );
}
}
jhs.cpp 소스코드 (메인파일)
#include "dp.h"
#include <iostream>
using namespace std;
#include <iostream>
using namespace std;
typedef struct Man // Sitdown Man Information Struct
{
int id;
Dining_Philosopher *dp;
HANDLE left,right;
} Man;
{
int id;
Dining_Philosopher *dp;
HANDLE left,right;
} Man;
DWORD WINAPI WriteToDatabase( LPVOID );
void main() // 5 is Maz Size
{
HANDLE ghMutex[5];
HANDLE aThread[5];
DWORD ThreadID;
struct Man DP[5];
int i,j,temp;
bool random_check; // If Event Random is coming, Same Number is interception
for(i=0;i<5;i++) // Create a mutex with no initial owner
{
ghMutex[i] = CreateMutex(
NULL, // default security attributes
FALSE, // initially not owned
NULL); // unnamed mutex
{
HANDLE ghMutex[5];
HANDLE aThread[5];
DWORD ThreadID;
struct Man DP[5];
int i,j,temp;
bool random_check; // If Event Random is coming, Same Number is interception
for(i=0;i<5;i++) // Create a mutex with no initial owner
{
ghMutex[i] = CreateMutex(
NULL, // default security attributes
FALSE, // initially not owned
NULL); // unnamed mutex
if (ghMutex == NULL)
{
printf("CreateMutex error: %d\n", GetLastError());
return;
}
}
{
printf("CreateMutex error: %d\n", GetLastError());
return;
}
}
// Setting each Man Number, Setting Left, Right
srand( (unsigned int)time(NULL) );
for(i=0;i<5;i++)
{
while(1)
{
temp=rand()%5+1;
random_check=true;
srand( (unsigned int)time(NULL) );
for(i=0;i<5;i++)
{
while(1)
{
temp=rand()%5+1;
random_check=true;
for(j=0;j<5;j++)
{
if(temp==DP[j].id) random_check=false;
}
if(random_check==true)
{
DP[i].id=temp;
DP[i].dp= new Dining_Philosopher(temp);
DP[i].right = ghMutex[i];
if(i==0) DP[i].left=ghMutex[4];
else DP[i].left=ghMutex[i-1];
break;
}
}
}
{
if(temp==DP[j].id) random_check=false;
}
if(random_check==true)
{
DP[i].id=temp;
DP[i].dp= new Dining_Philosopher(temp);
DP[i].right = ghMutex[i];
if(i==0) DP[i].left=ghMutex[4];
else DP[i].left=ghMutex[i-1];
break;
}
}
}
// Create worker threads
for( i=0; i < 5; i++ )
{
aThread[i] = CreateThread(
NULL, // default security attributes
0, // default stack size
(LPTHREAD_START_ROUTINE) WriteToDatabase,
&DP[i], // thread function arguments
0, // default creation flags
&ThreadID); // receive thread identifier
for( i=0; i < 5; i++ )
{
aThread[i] = CreateThread(
NULL, // default security attributes
0, // default stack size
(LPTHREAD_START_ROUTINE) WriteToDatabase,
&DP[i], // thread function arguments
0, // default creation flags
&ThreadID); // receive thread identifier
if( aThread[i] == NULL ){
printf("CreateThread error: %d\n", GetLastError());
return;
}
}
printf("CreateThread error: %d\n", GetLastError());
return;
}
}
// Wait for all threads to terminate
WaitForMultipleObjects(5, aThread, TRUE, INFINITE);
WaitForMultipleObjects(5, aThread, TRUE, INFINITE);
// Close thread and mutex handles
for( i=0; i < 5; i++ ) CloseHandle(aThread[i]);
for( i=0; i < 5; i++ ) CloseHandle(ghMutex[i]);
}
for( i=0; i < 5; i++ ) CloseHandle(aThread[i]);
for( i=0; i < 5; i++ ) CloseHandle(ghMutex[i]);
}
DWORD WINAPI WriteToDatabase( LPVOID lpParam )
{
Man *temp = (Man *)lpParam;
DWORD dwCount=0, LWait,RWait;
{
Man *temp = (Man *)lpParam;
DWORD dwCount=0, LWait,RWait;
//If Eating Counting Numbers are 5, End
while( dwCount < 5 )
{
// Waiting eat
temp->dp->think();
// Waiting Part. Ror Left and Right Catching
LWait=WaitForSingleObject(temp->left,INFINITE);
RWait=WaitForSingleObject(temp->right,INFINITE);
while( dwCount < 5 )
{
// Waiting eat
temp->dp->think();
// Waiting Part. Ror Left and Right Catching
LWait=WaitForSingleObject(temp->left,INFINITE);
RWait=WaitForSingleObject(temp->right,INFINITE);
switch(LWait & RWait)
{
// If left and right empty
case WAIT_OBJECT_0: __try
{
temp->dp->eat();
dwCount++;
}
__finally
{
ReleaseMutex(temp->left);
ReleaseMutex(temp->right);
}
break;
case WAIT_ABANDONED:
return FALSE;
}
}
return TRUE;
}
{
// If left and right empty
case WAIT_OBJECT_0: __try
{
temp->dp->eat();
dwCount++;
}
__finally
{
ReleaseMutex(temp->left);
ReleaseMutex(temp->right);
}
break;
case WAIT_ABANDONED:
return FALSE;
}
}
return TRUE;
}
이건 실행화면